Mastering Django Query Filters: A Comprehensive Guide to lte
, lt
, in
, contains
, and More.
Django, the high-level Python web framework, is renowned for its ability to simplify database interactions through its Object-Relational Mapping (ORM) layer. One of the most powerful features of Django’s ORM is its query filtering capabilities. In this article, we’ll dive deep into some of the most commonly used query filters such as lte
, lt
, in
, contains
, and more. By the end of this guide, you'll have a solid understanding of how to leverage these filters to write efficient and readable database queries.
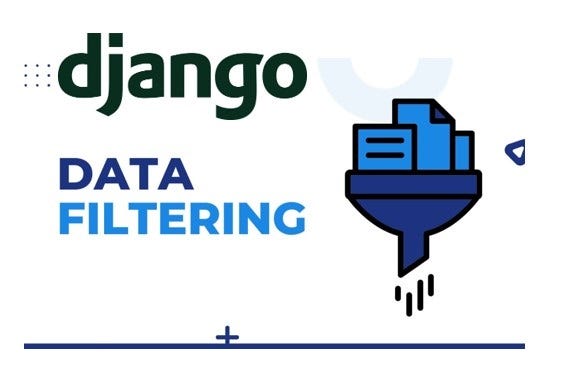
Introduction to Django Query Filters
Django’s ORM allows you to interact with your database using Python code instead of writing raw SQL queries. Query filters are methods that you can chain onto a QuerySet to narrow down the results based on specific conditions. These filters are translated into SQL queries under the hood, making it easier to work with databases in a Pythonic way.
Basic Filtering with filter()
and exclude()
Before diving into specific filters, let’s start with the basics. The filter()
method is used to retrieve objects that match certain criteria, while exclude()
does the opposite—it excludes objects that match the criteria.
from myapp.models import Article
# Retrieve all articles with a status of 'published'
published_articles = Article.objects.filter(status='published')
# Exclude articles with a status of 'draft'
non_draft_articles = Article.objects.exclude(status='draft')
Using lte
and lt
for Range Queries
When you need to filter results based on a range of values, Django provides the lte
(less than or equal to) and lt
(less than) filters. These are particularly useful when working with numeric fields or dates.
from myapp.models import Product
# Retrieve products with a price less than or equal to 100
cheap_products = Product.objects.filter(price__lte=100)
# Retrieve products with a price less than 50
very_cheap_products = Product.objects.filter(price__lt=50)
Similarly, you can use gte
(greater than or equal to) and gt
(greater than) for the opposite conditions.
# Retrieve products with a price greater than or equal to 200
expensive_products = Product.objects.filter(price__gte=200)
# Retrieve products with a price greater than 500
very_expensive_products = Product.objects.filter(price__gt=500)
Filtering with in
for Multiple Values
The in
filter allows you to specify multiple values for a field. This is useful when you want to retrieve objects that match any of the specified values.
from myapp.models import Customer
# Retrieve customers from specific cities
customers_in_cities = Customer.objects.filter(city__in=['New York', 'Los Angeles', 'Chicago'])
This query will return all customers whose city
field is either 'New York', 'Los Angeles', or 'Chicago'.
Searching with contains
, icontains
, startswith
, and endswith
Django provides several filters for searching text fields:
contains
: Case-sensitive substring search.icontains
: Case-insensitive substring search.startswith
: Case-sensitive search for fields that start with a specific value.istartswith
: Case-insensitive search for fields that start with a specific value.endswith
: Case-sensitive search for fields that end with a specific value.iendswith
: Case-insensitive search for fields that end with a specific value.
from myapp.models import BlogPost
# Retrieve blog posts that contain the word 'Django' in the title
django_posts = BlogPost.objects.filter(title__contains='Django')
# Case-insensitive search for blog posts starting with 'django'
django_posts_case_insensitive = BlogPost.objects.filter(title__istartswith='django')
The icontains
filter is particularly useful when you want to ensure that your search is not case-sensitive, making it more user-friendly.
Filtering with isnull
for Null Values
The isnull
filter is used to check if a field is NULL
or not.
from myapp.models import Employee
# Retrieve employees without a manager (manager field is NULL)
employees_without_manager = Employee.objects.filter(manager__isnull=True)
# Retrieve employees with a manager (manager field is not NULL)
employees_with_manager = Employee.objects.filter(manager__isnull=False)
Date and Time Filters
Django provides a variety of filters for working with date and time fields:
date
: Filters by a specific date.year
,month
,day
: Filters by year, month, or day.hour
,minute
,second
: Filters by hour, minute, or second.range
: Filters within a date or time range.
from myapp.models import Event
from datetime import date, timedelta
# Retrieve events happening in 2023
events_2023 = Event.objects.filter(date__year=2023)
# Retrieve events happening today
today = date.today()
events_today = Event.objects.filter(date__date=today)
# Retrieve events happening in the next 7 days
next_week = today + timedelta(days=7)
upcoming_events = Event.objects.filter(date__range=[today, next_week])
Combining Filters with Q
Objects
Sometimes, you may need to combine multiple filters using logical operators like AND
or OR
. Django's Q
objects allow you to build complex queries with ease.
from django.db.models import Q
from myapp.models import Article
# Retrieve articles that are either published or have a high priority
articles = Article.objects.filter(Q(status='published') | Q(priority='high'))
# Retrieve articles that are published and have a high priority
articles = Article.objects.filter(Q(status='published') & Q(priority='high'))
You can also use the ~
operator to negate a Q
object, effectively creating a NOT
condition.
# Retrieve articles that are not published
non_published_articles = Article.objects.filter(~Q(status='published'))
Conclusion
Django’s query filtering capabilities are both powerful and flexible, allowing you to write complex database queries with minimal effort. By mastering filters like lte
, lt
, in
, contains
, isnull
…etc. And combining them with Q
objects, you can efficiently retrieve the data you need while keeping your code clean and readable.
Whether you’re building a simple blog or a complex e-commerce platform, understanding these filtering techniques will significantly enhance your ability to work with Django’s ORM. So go ahead, experiment with these filters, and take your Django skills to the next level!